Have you just installed Google AdSense and discovered your site is broken?
We recently installed Google AdSense on to a couple of e-commerce sites that we manage and very quickly found that our mobile skins were completely broken. On both sites, the web pages refused to scroll and a popup categories menu was displaying off screen and wasn’t even visible. Both sites were completely unusable. Whilst we were inspecting it carefully using Chrome Developer Tools, we noticed that the div heights were being dynamically overwritten by an unknown script. We eventually confirmed that the culprit was Google AdSense itself. In this article, we detail the process of how to resolve the issue.
Cause: Google AdSense is inserting code into your website!
Google AdSense will dynamically override and insert “height: auto !important;” into each of the div’s that it wants to show an advertisement in so that they can resize according to the size of the advertisement it wants to show on your site. This all makes sense. Unfortunately, it can totally destroy your website and make it unusable.
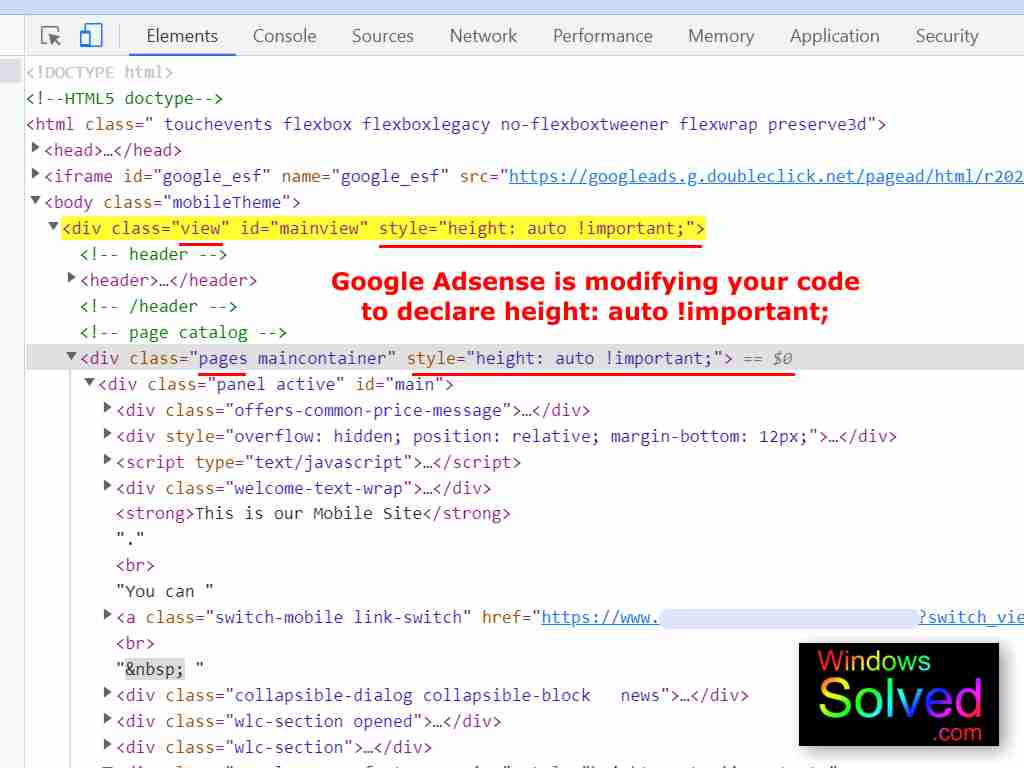
Our first attempt to solve this problem was to reset the div’s from overflow: hidden to overflow: visible. This will get your scroll working again but your content containers will be of the wrong size and menus and popups may not display where they should and need a lot of scrolling to see the content. We thought there had to be a better solution.
The solution to Google AdSense inserting “height: auto !important;” :
Firstly, we would like to credit the following pages for part of our solution.
https://weblog.west-wind.com/posts/2020/May/25/Fixing-Adsense-Injecting-height-auto-important-into-scrolled-Containers who pays credit to the following page in developing the code https://stackoverflow.com/questions/55695667/adsense-injecting-style-tag-into-my-page-in-chrome
We confirm that the Mutation Observer JavaScript code they have published does indeed work.
In their examples, they include script src=”https://pagead2.googlesyndication.com/pagead/js/adsbygoogle.js” async></script in the top. However, if you have AdSense installed on your site already, AdSense already includes the same line of code and Developer Console reports that it should only be included once per page. So in our code examples below, we have removed it, assuming you already have AdSense installed – hence why you are now reading this article!
We also found that the code only worked for modifying the “height: auto !important;” declaration for ONE div. In our case we had to fix 2 different divs to allow our webpages to scroll and menus to display correctly. This took quite some work to work it out, as Chrome console reports that only one instance of observe can run at a time. The solution for changing multiple divs is also below. Further, it is was also found that where you put this code on your site makes the difference between it working and not working. The sites we were working on were based on a PHP & Smarty Templates platform. The mobile skin was also using Flexbox and jQuery. Our solution worked in both the Flexbox & jQuery mobile skin, and also in the standard PHP & SMARTY main website. It is not dependent upon Flexbox to fix your AdSense problems.
The solution allows AdSense to modify your pages, load your ads and then a script is called to change your site markup back to enable it work properly. A great solution!
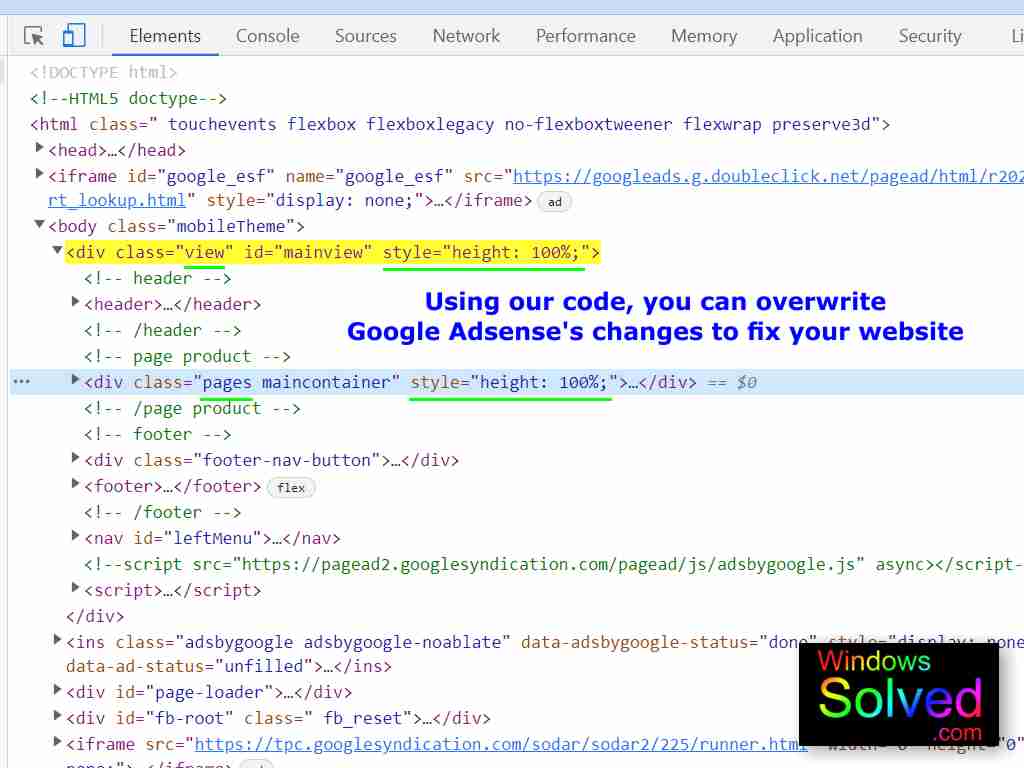
Step 1 : Identify the div/divs that AdSense is changing and causing your site to break.
Navigate your browser to a page that you are having trouble with and open Chrome Developer Console by hitting F12. Firefox and Opera appear to have the same developer console, so use which ever browser you like. You will want to be on the Elements tab.
Reload the page a few times and watch it carefully as it loads. You should see some of your div style height declarations being dynamically changed. You may also need to expand the sections as it all happens pretty quick. You might need to do it a few times on each div to check. You can cross check it against your own html or php code to confirm if AdSense has changed it. On each of the divs that you find style=”height: auto !important;”>, look in the elements Styles tab, and uncheck the box next to height auto !important declaration and test your site response. As you start to identify which divs need to be changed, keep narrowing it down until you find only the essential divs that need changing. In our case, we needed 2 on the mobile skin and 3 on parts of the desktop site. Once you have identified which exact divs need to be changed, proceed.
Step 2 : In the Divs that need to be changed, we need to look at the class=”….” but preferably an id=”…” for each div.
Using ID is preferable to using Class. A well marked up site will only a unique ID’s used for each section. Unlike IDs, css classes, can be used in multiple locations throughout your website. By using the following code with css classes, be sure to check through your pages to see if other parts of your site are also using the same css class. It will affect those classes too. If your html code doesn’t have an ID, we strongly recommend you assign one to avoid any unforeseen changes to other parts of your site. If you are confident that the css classes aren’t being used elsewhere, the code can be used with the class name. In the example picture above, the first div in yellow has a class of view and an ID of mainview. The second div in blue only has a multiple class declaration of pages & maincontainter. As we confirmed that css class isn’t used anywhere else, we implemented the code on classes. But we do recommend you assign IDs to be safer.
Step 3 – Creating your code to overwrite Google Adsense style=”height: auto !important;”
As our PHP sites used Smarty Templates, we created a file with the rest of the AdSense files so that it can just be called with {include file=”…”}. This will keep your templates clean and you can call the single file where ever you need it. If you need to change it later, you also only have to update 1 file instead of search through each of your pages to find and change each instance of the code.
You must have Google AdSense installed on your site for this code to work.
Be sure to also read our script notes below the script codes.
Code to modify 1 Div by Class Name (See script notes below)
<script>
// Authored & Published by https://windowssolved.com based on code from
// https://stackoverflow.com/questions/55695667/adsense-injecting-style-tag-into-my-page-in-chrome
// https://weblog.west-wind.com/posts/2020/May/25/Fixing-Adsense-Injecting-height-auto-important-into-scrolled-Containers
var change = document.getElementsByClassName('YOUR-DIV-CLASSNAME')[0];
const observer = new MutationObserver(function (mutations, observer) {
change.style.height = "100%"
});
observer.observe(change, {
attributes: true,
attributeFilter: ['style']
});
Code to modify 1 Div by ID (See script notes below)
<script>
// Authored & Published by https://windowssolved.com based on code from
// https://stackoverflow.com/questions/55695667/adsense-injecting-style-tag-into-my-page-in-chrome
// https://weblog.west-wind.com/posts/2020/May/25/Fixing-Adsense-Injecting-height-auto-important-into-scrolled-Containers
var change = document.getElementById('YOUR-DIV-ID-NAME')[0];
const observer = new MutationObserver(function (mutations, observer) {
change.style.height = "100%"
});
observer.observe(change, {
attributes: true,
attributeFilter: ['style']
});
</script>
Our code to modify 2 or more Divs by Class Name (See script notes below)
<script>
// Authored, Developed & Published by https://windowssolved.com based on code from
// https://stackoverflow.com/questions/55695667/adsense-injecting-style-tag-into-my-page-in-chrome
// https://weblog.west-wind.com/posts/2020/May/25/Fixing-Adsense-Injecting-height-auto-important-into-scrolled-Containers
var change = document.getElementsByClassName('YOUR-DIV-CLASSNAME-1')[0];
var change2 = document.getElementsByClassName('YOUR-DIV-CLASSNAME-2')[0];
const observer = new MutationObserver(function (mutations, observer) {
change.style.height = "100%"
change2.style.height = "100%"
});
observer.observe(change, {
attributes: true,
attributeFilter: ['style']
});
</script>
Our code to modify 2 or more Divs by ID (See script notes below)
<script>
// Authored, Developed & Published by https://windowssolved.com based on code from
// https://stackoverflow.com/questions/55695667/adsense-injecting-style-tag-into-my-page-in-chrome
// https://weblog.west-wind.com/posts/2020/May/25/Fixing-Adsense-Injecting-height-auto-important-into-scrolled-Containers
var change = document.getElementById('YOUR-DIV-ID-NAME-1')[0];
var change2 = document.getElementById('YOUR-DIV-ID-NAME-2')[0];
const observer = new MutationObserver(function (mutations, observer) {
change.style.height = "100%"
change2.style.height = "100%"
});
observer.observe(change, {
attributes: true,
attributeFilter: ['style']
});
</script>
Script Notes :
- The [0] at the end of the lines may or not work for you. Developer console shows a small ==$0 after the div line, indicating the DOM Node Index. We found that the [0] was required on our mobile skin. However, on our desktop site, the [0] had to be removed. If one way doesn’t work for you, try the other.
- If using ID, do NOT put the # in front of the ID name, just the name as shown in the code.
- The line change.style.height = “100%” – you can change 100% to whatever your site requires. You can even leave it blank such as “”. We tested it with one line at 100% and the other at 90% and both the divs were correctly changed to 100 & 90 as we specified.
- Do NOT include !important in your new height declarations. Firstly, it will stop the script working. Secondly, the !important is not required as the CSS has already been fully executed.
- The line observer.observe(change, { is only required once, even if using multiple variables for different divs. The script will change all of the var specified in lines 1 & 2, even though only one is specified in the observer.observe line.
- If you need more than 2 divs modified, add a new var change3 = document.getElementById(‘YOUR-DIV-ID-NAME-3’)[0]; after line 2 and then add change3.style.height = “100%” after line 5 etc.
- If using SMARTY Templates, make your you wrap your script in the {literal}yourscript{/literal} tags inside your tpl file.
Step 4 – Where to place the code in your site
This script needs to run after Google Adsense has made its changes. It needs to observe what changes are being made.
Do not put it in your head section – it won’t work even if you defer it.
For us, the best location to put the script was just before the closing body tag </body>. This gives time for the page to load and for AdSense to make its changes and then the script will change it again – all before the page has finished rendering and your scroll and menus should be immediately available. You may need to experiment with the location, depending on the location of the DIVs that Adsense is changing – just make sure it is AFTER your AdSense code!
Step 5 – Check your site in Developer Console
Reload your site in Developer Console and to see if the required changes have taken effect.
If the changes have not taken effect, please check your class names or IDs carefully, read our Script Notes in Step 3 and check Step 4 again. We have confirmed this works on multiple sites on different web servers.
![]() | If you found this article helpful, please leave us a comment and a rating below. You are also welcome to shout us a coffee by clicking on the cup! |
![]() | This solution was developed and confirmed in PHP 7.0.33 with Linux & Web server nginx/1.22.1 by windowssolved.com |
![]() | This is an original article copyright to windowssolved.com This article may be shared via link to this page. You may not repost the content of this page, even with attribution without prior written permission. |
0 Comments